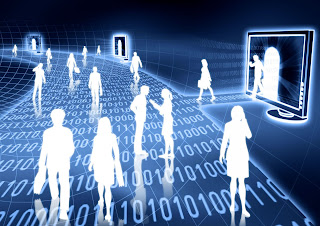
Definitions of Information technology (IT) on the Web:
Includes all matters concerned with the furtherance of computer science and technology and with the design, development, installation, and implementation of information systems and applications [San Diego State University]. An information technology architecture is an integrated framework for acquiring and evolving IT to achieve strategic goals. It has both logical and technical components. Logical components include mission, functional and information requirements, system configurations, and information flows. Technical components include IT standards and rules that will be used to implement the logical architecture.
For the user who wants to find an example of a specific process, such as "Outsourcing" or "Knowledge Management," regardless of how that process is used.
such as "Marketing," "Finance," "Manufacturing."
For the user who wants to find examples of innovative practices within these function areas, regardless of what process is used.
For the user who wants to find examples of organizations that are successfully "Innovative," "Productive" or "Agile," regardless of the process(es) they use to achieve that result.
For users interested in examples of a specific technology in use, such as the" Internet" or "Decision Support Systems."
Identifies whether the example is a documented "Success," a new "Experiment" of which the results are not yet known, or a "Failed Experiment," which is an example of a practice that didn't work.
Describes an idea that's more than a process or technology, such as "Electronic Market" or "Emerging Economies."
Organizations that are able to respond quickly to external change. (Result)
Refers to the speed of operations within an organization and speed in responding to customers (reduced cycle times). In contrast, "Adaptable" refers to the ability to respond to macro changes, such as a change in legislation, entrance of a strong competitor, etc. (Result)
Interesting contractual agreements with suppliers, distributors, customers, competitors, research organizations, joint ventures, government-industry partnerships, consortia. If no contract is involved, the term "collaboration" is used. (Process)
As distinct from "alliance," which is a contractual agreement, this term refers to an informal collaboration between a company and an outside entity such as a supplier, customer, even a competitor. When referring to internal collaborations, such as different teams or units of an organization working together, the term "Enterprise Integration" is used. (Process)
Describes an organization's anticipation or exploitation of an emerging consumer trend. (Concept)
A successful practice of continuous improvement, follow-on of TQM principles. (Process)
Coordination can refer to coordination in human systems, in parallel and distributed systems, and in complex systems that include both people and computers. The definition put forth by Malone and Crowston (1994) is: "Coordination is managing dependencies between activities." This definition is consistent with the simple intuition that, if there is no interdependence, there is nothing to coordinate. (Concept)
Computer Supported Cooperative Work, effective or novel uses of groupware. (Process)
The organization has built an interesting organizational culture, has a strong set of values. (Result)
An organizational structure in which decision-making authority is located not at the center but at the nodes. (Concept)
Information technology and software specifically designed to help people at all levels of the company (below the executive level) make decisions. This keyword is used when a company is making creative or extensive use of DSS, above and beyond typical practice. The term "Executive Information System" is a subset of Decision Support System because EIS focuses only on executives' (upper management's) use of strategic information and technology. (Technology)
Organizations that are using electronic markets as a way of coordinating information and the supply chain. New information technologies that enable the creation of new electronic marketplaces, use of electronic brokers. Existing industry boundaries disappear and create new cross-industry markets. Companies operating across multiple value chains. (Concept)
Organizations operating in emerging economies such as Eastern Europe, Brazil, Malaysia, etc. (Concept)
Refers to internal coordination processes among different core activities. (Process)
Ways executives (only upper level management) are making use of strategic information and technology. This term is distinct from Decision Support System, which are systems used at all levels below the executive level to make decisions. (Technology)
The company is experimenting with a new practice, the results of which are not yet known. (Stage: as distinguished from a Success Story or a Failed Experiment.)
Interesting in that the practice or approach didn't work. (Stage: cf. Success Story and Experiment)
An organizational structure in which the center reserves some decision-making authority, but all decisions not specially reserved are made at the nodes. Decisions are made at the lowest appropriate level. (Concept)
The interesting feature is a new approach to finance. (Function)
A unique approach to planning, using scenarios, interesting use of information. Simply using new forecasting software is not interesting, unless it is used in a nontypical way. (Process)
As distinct from "Global Coordination," a "Global" organization is simply one which has sales internationally, but doesn't necessarily coordinate work globally. The company isn't a multinational company either, in that it doesn't have a big presence in multiple nations. Rather, the keyword "Global" denotes that the company is small but offers its products or services worldwide. (Concept)
An interesting process of coordination within a company across different countries. (Process)
Decision-making specifically by a group, not an individual. This group does not need to be a team. This keyword could be paired with CSCW when companies use computer networks/groupware to make decisions. If the decision is made by an individual, the keyword "Leadership" would be the term to use. The term "Decision Support System" should be used if the decision-making is based on the use of decision-support software. (Process or Concept)
An approach that has enabled the company to grow substantially in size or profits or market penetration. (Result)
Includes innovative approaches to compensation, pay for performance, flextime, benefits, stock plans, legal compliance, training. (Function)
A way of organizing start-up or young businesses to promote their growth. (Concept)
Refers to the dynamic movement of information through a company or to outside suppliers and customers. Firms may build direct linkages with their customers, suppliers, and partners. (Concept)
Includes both hardware and software. Use this term when the use of information technology is the underlying driver of the "interesting" feature or of the organization's profitability or productivity. This term can include computer modeling, simulation, innovative uses of A.I., automated knowledge discovery, data mining, data warehousing. (Technology)
Successful practices that lead to fast or fertile innovation and new product development. (Result)
Consultants who make their living doing short term projects. These companies are 100% Virtual Organizations. (Source of term: Thomas Malone and John Rockart in "Computers, Networks and the Corporation" Scientific American, Sept. 1991.) (Concept)
Organizations using the Internet in innovative ways. (Technology)
A company that makes its money by selling its knowledge. Companies with this keyword only sell the knowledge -- not knowledge embodied in a product or even a service. Instead, they sell their know-how or advice, such as the "answer networks" phenomenon predicted by Thomas Malone and John Rockart in "Computers, Networks and the Corporation" Scientific American, Sept. 1991. (Concept)
The way a company stores, organizes and accesses internal and external information. Narrower terms are: "Organizational Memory" and "Knowledge Transfer." (Process)
Effective sharing of ideas, knowledge, or experience between units of a company or from a company to its customers. The knowledge can be either tangible or intangible. (Process)
Ways management achieves oversight of projects, such as activity-based accounting. (Process)
The interesting feature is a new approach to manufacturing. (Function)
The interesting feature is a new approach to marketing. (Function)
Ways of achieving customization of product or service down to a run of one. (Concept)
Methods that a company has come up with to measure something, like effectiveness of a training program, IT productivity, customer satisfaction. (Concept)
Term referring to a type of organizational structure: refers to coordination beyond the company boundaries. (Concept)
An organization not based in the United States. (Concept)
Interesting ways of spotting opportunities or trends. (Process)
Companies that are undergoing or that have undergone a transformation. This keyword should always be used in conjunction with "Success Story" or "Experiment" or "Failed Experiment." (Process)
Based on Peter Senge's Fifth Discipline. (Process)
The way the company stores and keeps track of what it knows, company procedures, employees' skills. (Process)
Use of a new organizational form. An overall way to identify many examples of organizational structures. (Concept)
Companies outsourcing different functions. (Process)
This term is used to call attention to a very interesting process (especially for linkage to the Process Handbook.) (Process)
The company has found a practice that has succeeded in improving the productivity of the company. (Result)
A practice that relates only and directly to Research & Development. (Function)
A unique way of deploying resources. This keyword can include an "internal market" system in which employees within a company bid to work on a project, as opposed to a supervisor allocating their time. (Process)
Often used in conjunction with team-based. Differs from "Self-Organizing" in that a team may manage itself but it may have been brought together (organized) by someone else. (Concept)
Examples of self-organizing behavior, such as people joining around an idea like constructing a piece of software without centralized control. (Concept)
A unit or division of a company that is "spun off" (i.e., given complete independence to operate as its own for-profit company.) The spin-off company's stock may be publicly traded, and the parent company usually owns a percentage of the stock. (Process)
An interesting strategic approach or idea. (Function)
An example of a successful practice -- the company has achieved documented successful results. (Stage: This term is used to distinguish those companies that have succeeded with a practice from those that are experimenting (cf. "Experiment") with one or a documented failure (cf. "Failed Experiment"))
Methods of coordination and integration of processes within a traditional supply chain. Includes interesting practices regarding customers and suppliers, such as customers becoming co-producers (Alvin Toffler's term "prosumer.") (Process)
A management process of using teams. A team is together for a period of time and has shared goals, unlike a "group" which is simply a collection of individuals. (Process)
The ability to take a concept from outside the organization (typically from a government or university research programs) and create a product from it. (Process)
This may be a subgroup of Human Resource Policy. (To be decided whether it should be a separate keyword.)
A company that does not have a physical location. Rather, it is more like a collection of individuals that work from their home offices. (We may want to add the term "Virtual Team" to capture the essence of the companies like Reuters that create a team of people who are actually employed by other organizations but are brought together on a specific project.) (Concept)
The company has a physical location, but employees have no assigned offices. Employees may have lockers and "check out" a desk for the day, they may set up an "office" in their hotel, etc. This term is distinct from "Virtual Company" which refers to companies that have no one physical location at all, no collection of inventory that's held by the company, etc. (Concept)
The interesting feature is the nature of the organization's workforce population, such as working mothers, monks, etc. (Process)